π Playwright for 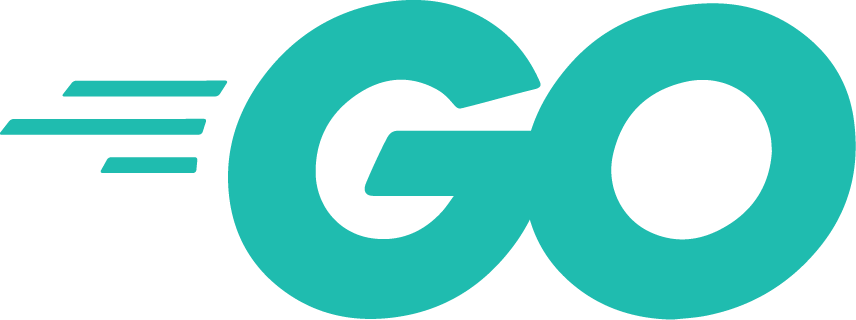
Looking for maintainers and see here. Thanks!
API reference | Example recipes |
Playwright is a Go library to automate Chromium, Firefox and WebKit with a single API. Playwright is built to enable cross-browser web automation that is ever-green, capable, reliable and fast.
Β | Linux | macOS | Windows |
---|---|---|---|
Chromium 134.0.6998.35 | β | β | β |
WebKit 18.4 | β | β | β |
Firefox 135.0 | β | β | β |
Headless execution is supported for all the browsers on all platforms.
Installation
go get -u github.com/playwright-community/playwright-go
Install the browsers and OS dependencies:
go run github.com/playwright-community/playwright-go/cmd/playwright@latest install --with-deps
# Or
go install github.com/playwright-community/playwright-go/cmd/playwright@latest
playwright install --with-deps
Alternatively you can do it inside your program via which downloads the driver and browsers:
err := playwright.Install()
Capabilities
Playwright is built to automate the broad and growing set of web browser capabilities used by Single Page Apps and Progressive Web Apps.
- Scenarios that span multiple page, domains and iframes
- Auto-wait for elements to be ready before executing actions (like click, fill)
- Intercept network activity for stubbing and mocking network requests
- Emulate mobile devices, geolocation, permissions
- Support for web components via shadow-piercing selectors
- Native input events for mouse and keyboard
- Upload and download files
Example
The following example crawls the current top voted items from Hacker News.
package main
import (
"fmt"
"log"
"github.com/playwright-community/playwright-go"
)
func main() {
pw, err := playwright.Run()
if err != nil {
log.Fatalf("could not start playwright: %v", err)
}
browser, err := pw.Chromium.Launch()
if err != nil {
log.Fatalf("could not launch browser: %v", err)
}
page, err := browser.NewPage()
if err != nil {
log.Fatalf("could not create page: %v", err)
}
if _, err = page.Goto("https://news.ycombinator.com"); err != nil {
log.Fatalf("could not goto: %v", err)
}
entries, err := page.Locator(".athing").All()
if err != nil {
log.Fatalf("could not get entries: %v", err)
}
for i, entry := range entries {
title, err := entry.Locator("td.title > span > a").TextContent()
if err != nil {
log.Fatalf("could not get text content: %v", err)
}
fmt.Printf("%d: %s\n", i+1, title)
}
if err = browser.Close(); err != nil {
log.Fatalf("could not close browser: %v", err)
}
if err = pw.Stop(); err != nil {
log.Fatalf("could not stop Playwright: %v", err)
}
}
Docker
Refer to the Dockerfile.example to build your own docker image.
More examples
- Refer to helper_test.go for End-To-End testing
- Downloading files
- End-To-End testing a website
- Executing JavaScript in the browser
- Emulate mobile and geolocation
- Parallel scraping using a WaitGroup
- Rendering a PDF of a website
- Scraping HackerNews
- Take a screenshot
- Record a video
- Monitor network activity
How does it work?
Playwright is a Node.js library which uses:
- Chrome DevTools Protocol to communicate with Chromium
- Patched Firefox to communicate with Firefox
- Patched WebKit to communicate with WebKit
These patches are based on the original sources of the browsers and donβt modify the browser behaviour so the browsers are basically the same (see here) as you see them in the wild. The support for different programming languages is based on exposing a RPC server in the Node.js land which can be used to allow other languages to use Playwright without implementing all the custom logic:
The bridge between Node.js and the other languages is basically a Node.js runtime combined with Playwright which gets shipped for each of these languages (around 50MB) and then communicates over stdio to send the relevant commands. This will also download the pre-compiled browsers.
Is Playwright for Go ready?
We are ready for your feedback, but we are still covering Playwright Go with the tests.